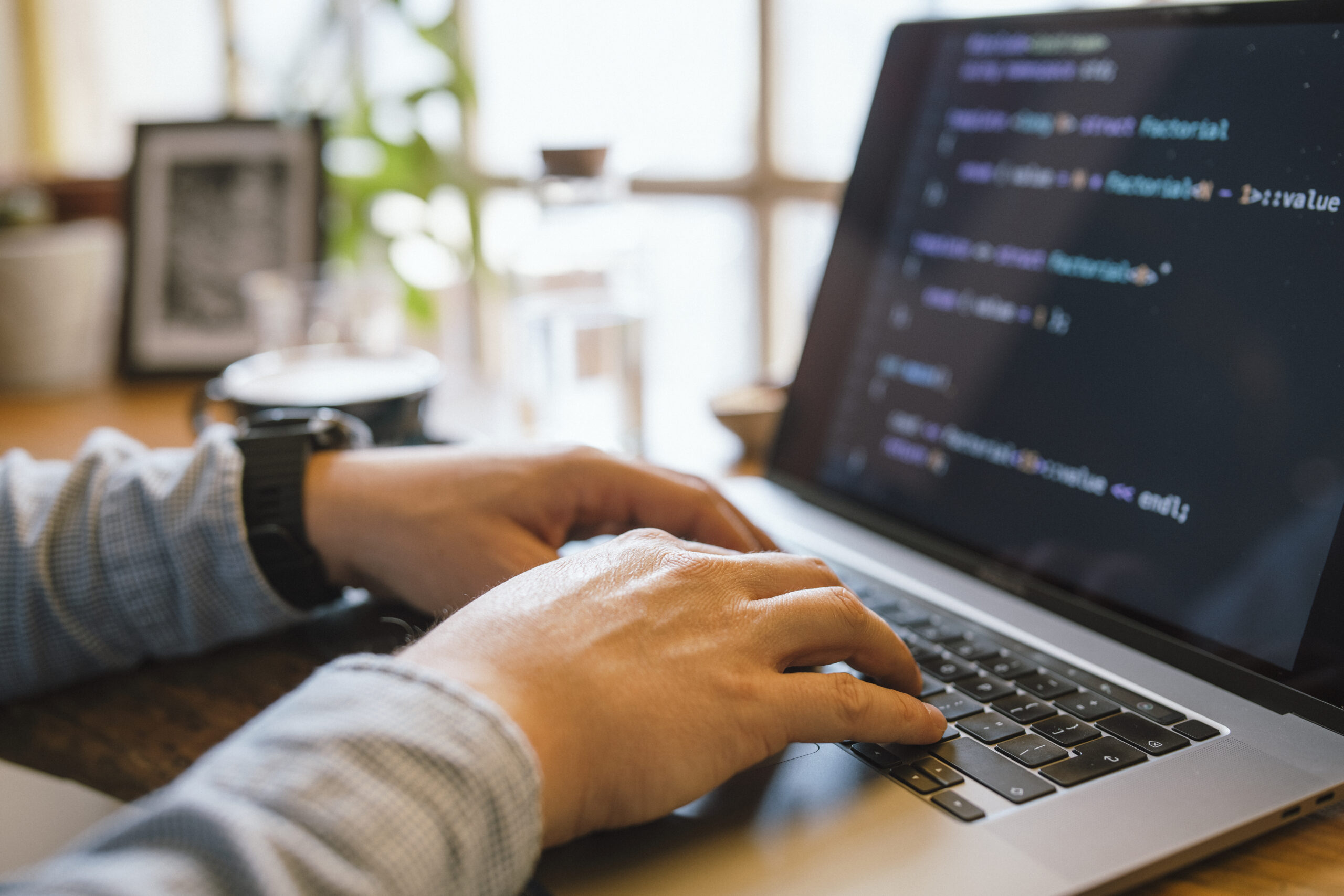
Debugging is Among the most essential — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a novice or even a seasoned developer, sharpening your debugging expertise can help save several hours of annoyance and radically help your efficiency. Here i will discuss quite a few procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Equipment
One of several quickest techniques developers can elevate their debugging abilities is by mastering the resources they use each day. Whilst creating code is 1 part of enhancement, figuring out the way to communicate with it successfully during execution is Similarly critical. Present day growth environments come Geared up with strong debugging capabilities — but many builders only scratch the surface area of what these applications can perform.
Consider, for example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code within the fly. When made use of accurately, they let you observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, perspective genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn annoying UI challenges into manageable jobs.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle about running processes and memory management. Mastering these tools might have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic modifications.
In the end, mastering your equipment suggests likely further than default configurations and shortcuts — it’s about acquiring an personal expertise in your development atmosphere in order that when concerns come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to devote solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and infrequently neglected — methods in successful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to create a dependable natural environment or circumstance exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of prospect, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Ask issues like: What actions triggered The problem? Which atmosphere was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it gets to isolate the exact problems below which the bug takes place.
When you’ve gathered sufficient information and facts, make an effort to recreate the problem in your local ecosystem. This might necessarily mean inputting the identical details, simulating equivalent person interactions, or mimicking method states. If The difficulty seems intermittently, contemplate crafting automated assessments that replicate the sting instances or condition transitions associated. These tests not simply help expose the trouble but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than specific configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It calls for endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. With a reproducible scenario, You should use your debugging resources a lot more properly, examination likely fixes safely and securely, and converse far more Plainly with the staff or people. It turns an summary grievance into a concrete challenge — Which’s where by builders prosper.
Read through and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then observing them as annoying interruptions, developers ought to learn to take care of mistake messages as direct communications from the procedure. They generally inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in full. Quite a few developers, specially when underneath time stress, look at the primary line and instantly get started generating assumptions. But deeper from the error stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it issue to a particular file and line selection? What module or purpose triggered it? These issues can manual your investigation and position you toward the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is Among the most impressive tools in a developer’s debugging toolkit. When utilized successfully, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
A great logging technique starts with knowing what to log and at what amount. Popular logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard functions (like productive begin-ups), WARN for opportunity challenges that don’t crack the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Involve context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially valuable in creation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Using a very well-thought-out logging technique, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To correctly identify and resolve bugs, developers have to solution the procedure like a detective solving a mystery. This mentality allows break down advanced challenges into manageable components and comply with clues logically to uncover the foundation induce.
Start by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or functionality difficulties. Identical to a detective surveys against the law scene, collect as much appropriate facts as you could with out jumping to conclusions. Use logs, check circumstances, and consumer studies to piece jointly a transparent photo of what’s taking place.
Following, sort hypotheses. Check with on your own: What may very well be triggering this conduct? Have any adjustments not too long ago been produced towards the codebase? Has this problem occurred right before less than identical situation? The purpose is always to narrow down possibilities and identify opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the trouble in a managed setting. Should you suspect a specific purpose or part, isolate it and verify if The difficulty persists. Like a detective conducting interviews, ask your code issues and Allow the effects direct you closer to the reality.
Spend shut consideration to little aspects. Bugs typically hide from the least predicted locations—similar to a missing semicolon, an off-by-a person error, or simply a race problem. Be thorough and individual, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Just as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more effective at uncovering hidden difficulties in complex methods.
Publish Assessments
Crafting tests is one of the best strategies to transform your debugging skills and General advancement effectiveness. Assessments don't just help capture bugs early but also serve as a safety net that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with unit checks, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Each time a check fails, you instantly know exactly where to look, significantly lessening some time expended debugging. Unit tests are Particularly useful for catching regression bugs—challenges that reappear immediately after Earlier getting fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that various aspects of your software function alongside one another efficiently. They’re specifically useful for catching bugs that come about in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can tell you which Component of the pipeline failed and underneath what situations.
Writing assessments also forces you to Consider critically about your code. To check a feature adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In brief, producing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the trouble—watching your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your mind, reduce aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma when they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy and also a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
For those who’re caught, a good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute split. Use that time to move all-around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really really causes quicker and more practical debugging in the long run.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Every bug you come across is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or comprehension and allow you to Create more robust coding behavior relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Particularly powerful. Irrespective of whether it’s read more by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other individuals avoid the similar concern boosts team effectiveness and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. All things considered, some of the finest developers will not be the ones who publish perfect code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a new layer to the talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills will take time, observe, and patience — nevertheless the payoff is big. It makes you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.